[VB.NET] REST API⑨ Web APIからGETして一覧表示#1(Controller追加)
ビュー用のコントローラーを追加
まずはビュー用のコントローラを追加します。
ソリューションエクスプローラで、「Controllers」を右クリックして表示されるメニューから、
「追加」>「コントローラー」を選択します。
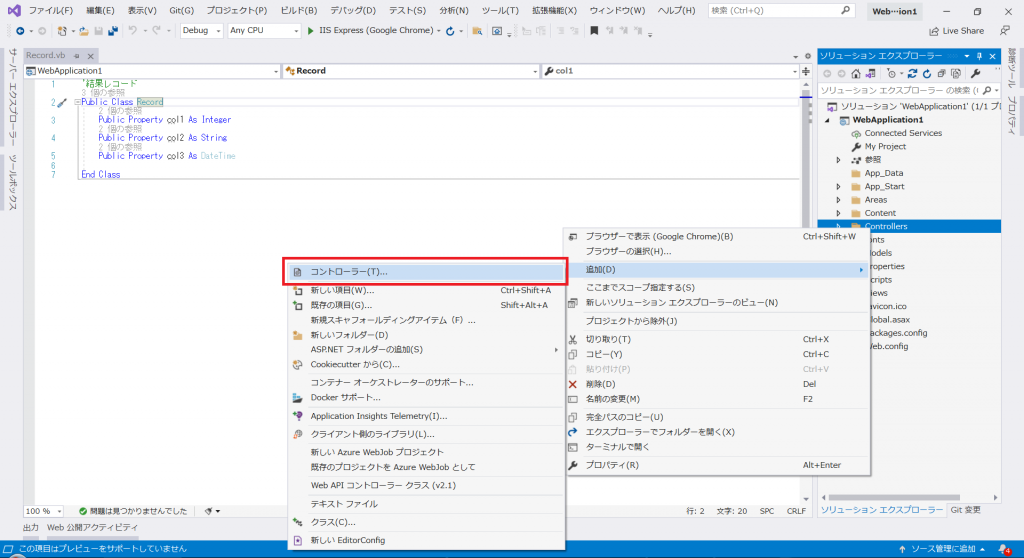
この例では、「MVC」>「コントローラー」から、
「読み取り/書き込みアクションがあるMVC 5」コントローラー」を選択し、「追加」をクリックします。
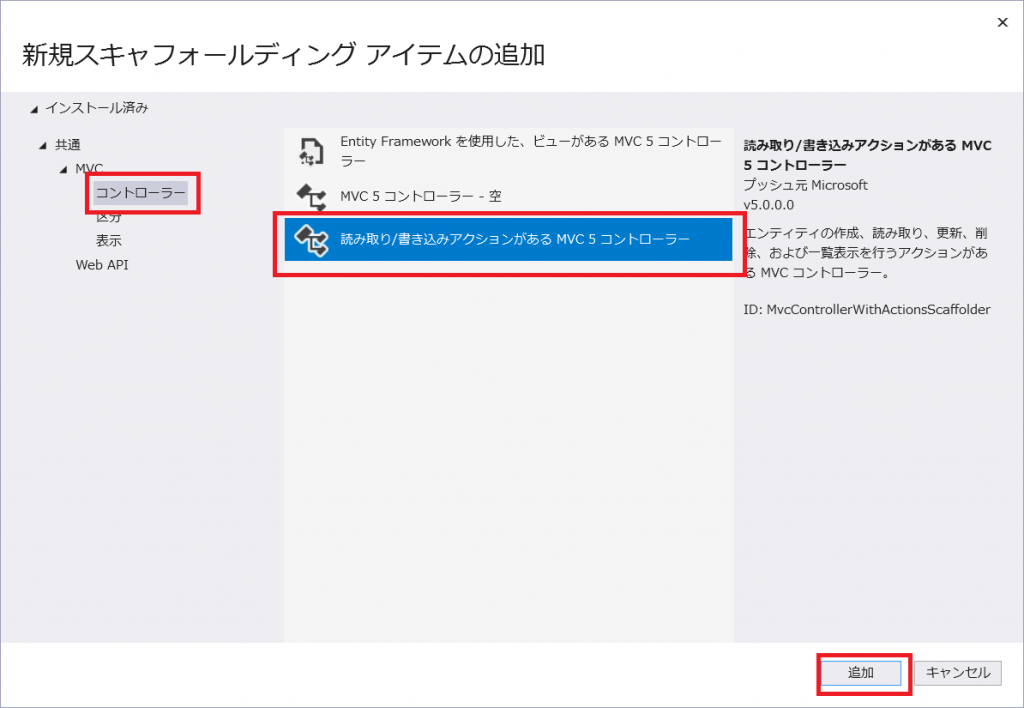
コントローラーの名前を入力して、「追加」をクリックします。
名前は必ず「***Controller」としてください。 ※「***」の部分は任意です。
この例では、「ViewTestController 」としています。
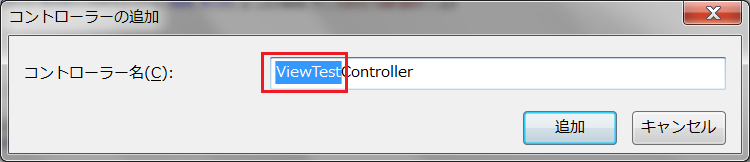
コントローラがー追加されます。
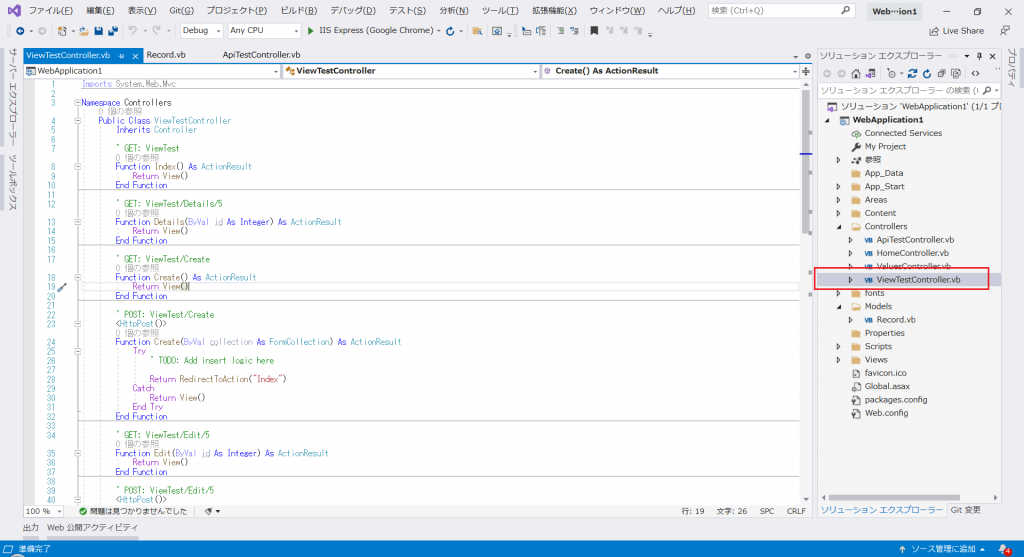
以下のようなコードが書かれています。
Imports System.Web.Mvc
Namespace Controllers
Public Class ViewTestController
Inherits Controller
' GET: ViewTest
Function Index() As ActionResult
Return View()
End Function
' GET: ViewTest/Details/5
Function Details(ByVal id As Integer) As ActionResult
Return View()
End Function
' GET: ViewTest/Create
Function Create() As ActionResult
Return View()
End Function
' POST: ViewTest/Create
<HttpPost()>
Function Create(ByVal collection As FormCollection) As ActionResult
Try
' TODO: Add insert logic here
Return RedirectToAction("Index")
Catch
Return View()
End Try
End Function
' GET: ViewTest/Edit/5
Function Edit(ByVal id As Integer) As ActionResult
Return View()
End Function
' POST: ViewTest/Edit/5
<HttpPost()>
Function Edit(ByVal id As Integer, ByVal collection As FormCollection) As ActionResult
Try
' TODO: Add update logic here
Return RedirectToAction("Index")
Catch
Return View()
End Try
End Function
' GET: ViewTest/Delete/5
Function Delete(ByVal id As Integer) As ActionResult
Return View()
End Function
' POST: ViewTest/Delete/5
<HttpPost()>
Function Delete(ByVal id As Integer, ByVal collection As FormCollection) As ActionResult
Try
' TODO: Add delete logic here
Return RedirectToAction("Index")
Catch
Return View()
End Try
End Function
End Class
End Namespace
コードを以下のように書き換えます。
この例では、HttpClientとHttpResponseMessageを使用してAPIにリクエストしています。
取得した結果は、JsonConvertを使ってJsonからList(Of Record)に変換しています。
変換後、「Return View(model) 」の部分でデータをビューに渡すようにしています。
Imports System.Web.Mvc
Namespace Controllers
Public Class ViewTestController
Inherits Controller
' GET: ViewTest
Function Index() As ActionResult
'一覧を取得するAPIのURL
Dim url As String
url = DirectCast(Request, System.Web.HttpRequestWrapper).Url.GetLeftPart(UriPartial.Authority) & "/api/ApiTest"
'GETを実行
Dim clt As New Net.Http.HttpClient
Dim result As New System.Net.Http.HttpResponseMessage
result = clt.GetAsync(url).Result
Dim model As List(Of Record)
If (result.StatusCode = Net.HttpStatusCode.OK) Then
'Jsonを取り出し
Dim json As String
json = result.Content.ReadAsStringAsync.Result
'デシリアライズ
model = Newtonsoft.Json.JsonConvert.DeserializeObject(Of List(Of Record))(json)
Else
model = New List(Of Record)
End If
Return View(model)
End Function
' GET: ViewTest/Details/5
Function Details(ByVal id As Integer) As ActionResult
Return View()
End Function
' GET: ViewTest/Create
Function Create() As ActionResult
Return View()
End Function
' POST: ViewTest/Create
<HttpPost()>
Function Create(ByVal collection As FormCollection) As ActionResult
Try
' TODO: Add insert logic here
Return RedirectToAction("Index")
Catch
Return View()
End Try
End Function
' GET: ViewTest/Edit/5
Function Edit(ByVal id As Integer) As ActionResult
Return View()
End Function
' POST: ViewTest/Edit/5
<HttpPost()>
Function Edit(ByVal id As Integer, ByVal collection As FormCollection) As ActionResult
Try
' TODO: Add update logic here
Return RedirectToAction("Index")
Catch
Return View()
End Try
End Function
' GET: ViewTest/Delete/5
Function Delete(ByVal id As Integer) As ActionResult
Return View()
End Function
' POST: ViewTest/Delete/5
<HttpPost()>
Function Delete(ByVal id As Integer, ByVal collection As FormCollection) As ActionResult
Try
' TODO: Add delete logic here
Return RedirectToAction("Index")
Catch
Return View()
End Try
End Function
End Class
End Namespace
まずは前準備としてビュー用のコントローラーを追加しました。
次回はこのコントローラーからデータを受け取って表示するビューを追加します。